I am accessing this website using a selenium chrome web driver, but whenever I submit the form it asks for me to verify that am not a bot. I have tried the following links (1, 2, 3) about avoiding detection but none of them worked.
public void AvoidDetectionChrome(){
System.setProperty("webdriver.chrome.driver", "path\\chromedriver.exe");
//Set ChromeOptions
ChromeOptions options = new ChromeOptions();
options.addArguments("start-maximized");
options.addArguments("--disable-blink-features=AutomationControlled");
options.setExperimentalOption("excludeSwitches", new String[]{"enable-automation"});
//Instantiate Web Driver
WebDriver driver = new ChromeDriver(options);
driver.get("https://www.edureka.co");
}
and code for Firefox driver:
public void AvoidDetectionFirefox(){
System.setProperty("webdriver.gecko.driver", "path\\geckodriver.exe");
//Set Firefox options
FirefoxOptions options = new FirefoxOptions();
options.addPreference("network.proxy.type", 1);
options.addPreference("network.proxy.http", "localhost");
options.addPreference("network.proxy.http_port", "3128");
options.addPreference("dom.webdriver.enabled", false);
options.setHeadless(false);
//Instantiate Web Driver
WebDriver driver = new FirefoxDriver(options);
driver.manage().window().maximize();
String url = "https://www.edureka.co";
driver.get(url);
}
Both of them fail to avoid the bot detection and I get asked confirmation once I click the submit button, as could be seen in the image below:
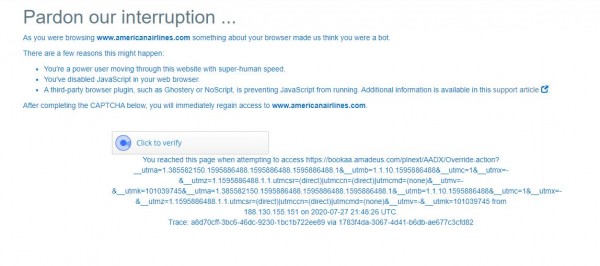
So how can I make any of the methods work when I submit the form and avoid the bot verification/detection?